Working with Arrays In JavaScript I (Adding, Removing, Combining and Finding Elements in an Array)
Working with Arrays In JavaScript I (Adding, Removing,Combining and Finding Elements in an Array)
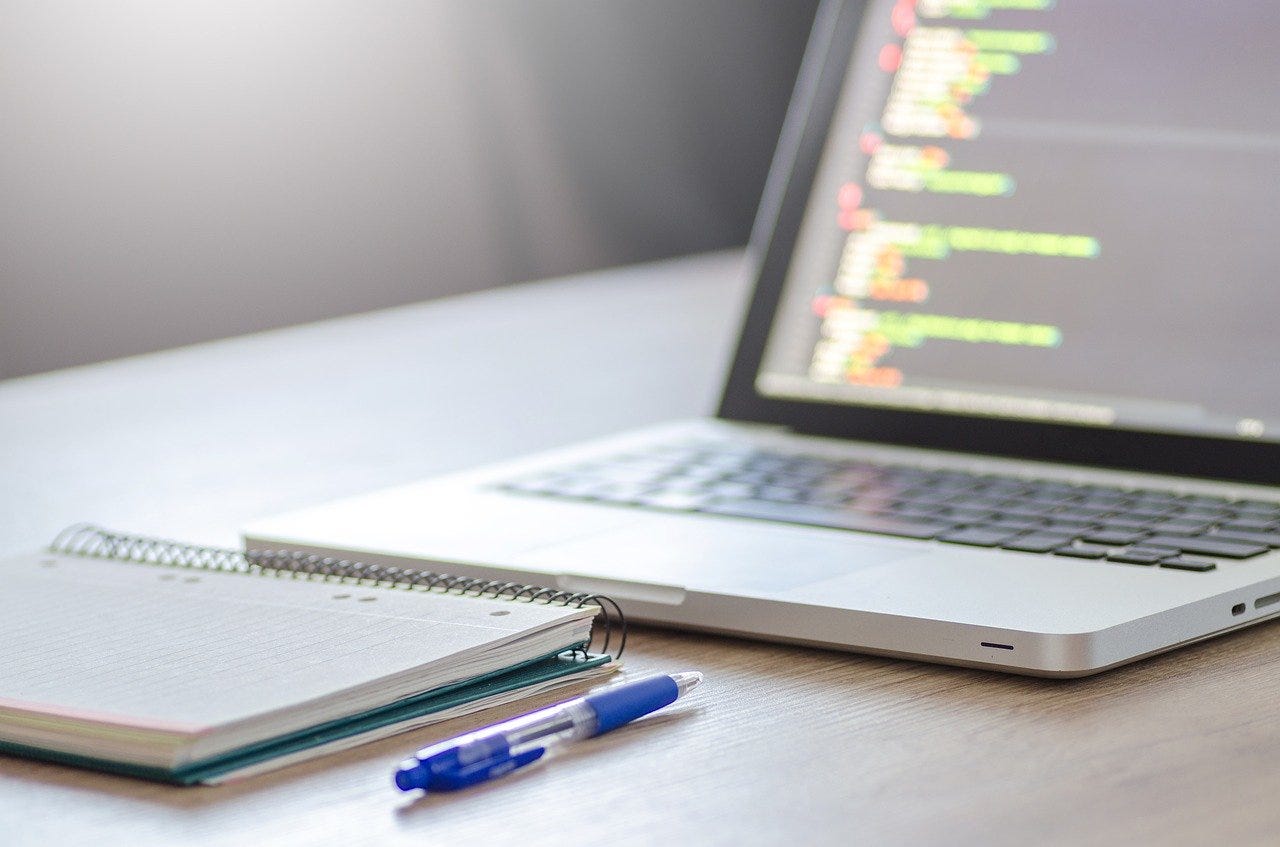
Arrays in JavaScript are Reference Types. It’s possible to modify the elements in a given array. Using the Dot Notation we can access all the properties and methods defined in an Array.
Adding Elements In An Array
It’s possible to modify an Array by Adding an element to it. We can add an element at the Beginning of an Array, In the Middle, and also at the End of an Array. We can do this using the following methods:
The push Method
The push Method allows us to add one or more element to the end of an array. Here’s an Example:
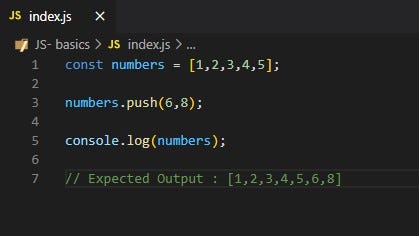
The unshift Method
The unshift method allows us to add an element at the beginning of an array. Here’s an Example:
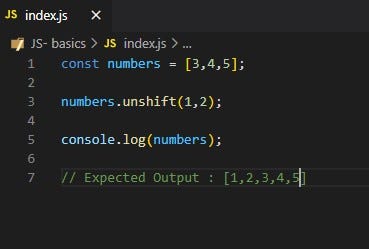
The Splice Method
With this method we can add an element at a given position. The first parameter in this method is our starting position, and the other parameter is for adding elements from our starting position. Here’s a clear example:
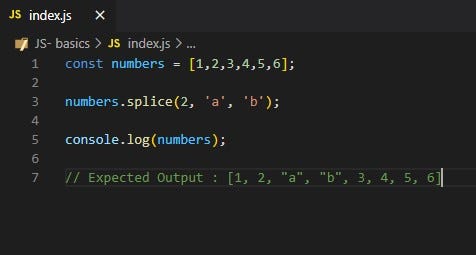
Removing Elements In An Array
Just as we can add an element to an array, it’s very possible to remove an element from the Beginning, in the middle and also at the end of an array. We can do this using the following methods:
The Pop Method
The pop method allows us to remove the last element in an array. Here’s an example:
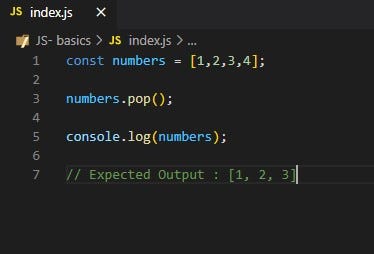
The Shift Method
Similar to the pop method,this method removes an element at the beginning of an array. Here’s an Example:
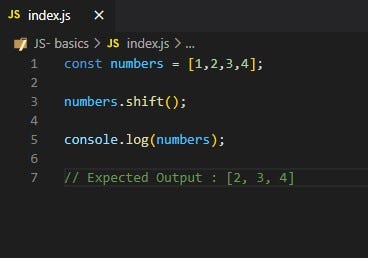
The Splice Method
With the splice method, we can pass the index of our starting index as the first parameter and the number of elements we want to remove as at the second parameter. Here’s a clear Example :
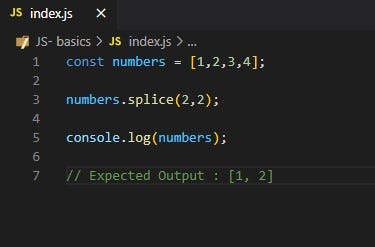
Finding Elements In an Array
Finding Elements really depends if we are storing either Primitive or Reference types in an Array.
Finding Elements in Primitive Types
Using The indexOf Method: With this method we can check to see if a certain element exists in an Array. If an Element exist in the array the console will return the index of the element on the console, and if the element does not exist it return -1. Here’s an example :
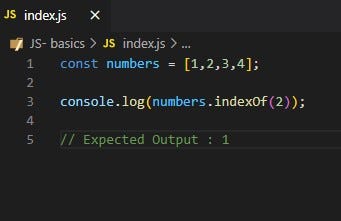
NOTE: The typeof element is really important, if we pass “1” as a string, the output will be -1 since we don’t “1” as a string in the array.
Using The lastIndexOf Method: This method will return the last index of a given element or -1 if the said element does not exist. Here’s an example:
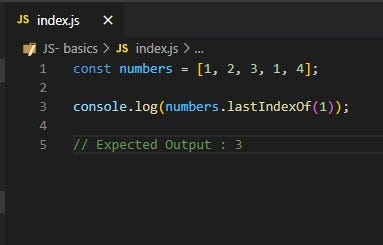
Using the includes Method: This Method checks if a given element exist in an array. If the output returns a boolean true, hence the element does exist in the given array.
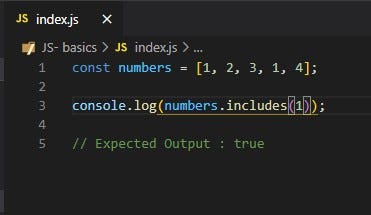
NOTE: There’s a second argument called fromIndex which is optional. The fromIndex is the index at which the search will begin. If the fromIndex is omitted, the search starts at 0.
Finding Elements in Reference Types
This is different from the Primitive type. Here there are methods for finding element which are Reference types which includes
The find Method: The Find method returns the value of the first element in the array that satisfies the provided testing functions. Otherwise undefined is returned. As an argument to the find method we need to pass a function. We call this function a Predicate or a Call-back function. It is used to determine if the given element exist in the array or not. Here’s an Example:
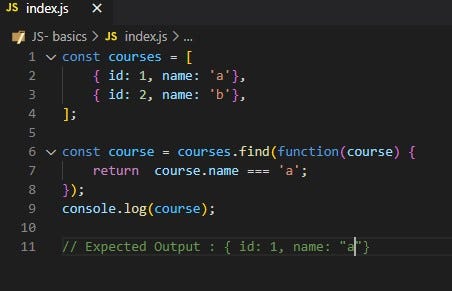
The findIndex Method: This works just like the find method, but this method returns the index of the element and if the element does not exist in the array it returns -1. Here’s an example:
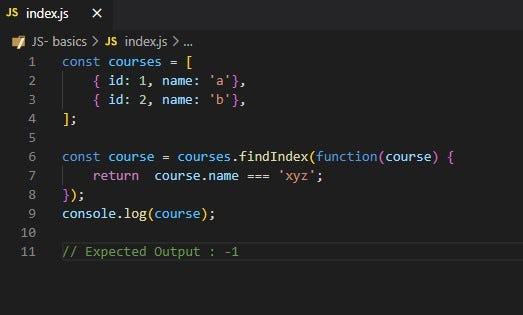
Combining Arrays
We can join two arrays together using the concat method. The concat method will basically concat two arrays and return a new array which will comprise of the two arrays elements. Let’s see an example:
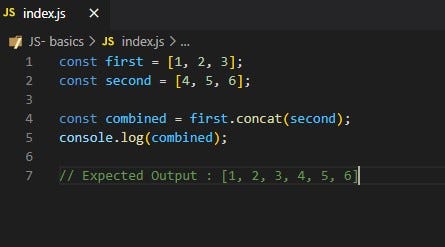
Thank You For Reading this Article, you can keep up with my progress by following me on my social media accounts.
Twitter: twitter.com/jhimmyofficial